I do not understand how to implement i2c coding for white box (ACK/NACK Bit) shown in image.
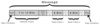
When writing to the slave, the master writes 8 bits of data (slave address) and select write mode
master must check ACK or NAK from the slave as it is writing bytes. If it receives ACK, it continues to send the next byte . If it receives NAK, then it must stop transmission and issue a stoP condition.
(if someone can help with rough code for explanation it would be appreciated )
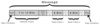
When writing to the slave, the master writes 8 bits of data (slave address) and select write mode
master must check ACK or NAK from the slave as it is writing bytes. If it receives ACK, it continues to send the next byte . If it receives NAK, then it must stop transmission and issue a stoP condition.
(if someone can help with rough code for explanation it would be appreciated )